In this ABAP article we learn how to define a local ABAP class. Local ABAP class will have class definition and class implementation. In class definition, define the components of the class, such as attributes, methods, events, constants, type, and implemented interfaces. Syntax to define class is:
CLASS <classname> DEFINTION.
//components of class
...
...
ENDCLASS.
Class Implementation is mandatory if methods are defined in class definition. In class implementation, we implement methods defined in class definition. Syntax for class implementation is:
CLASS <classname> IMPLEMENTATION.
METHOD <methodname1>.
...
ENDMETHOD.
METHOD <methodname2>.
...
ENDMETHOD.
...
...
ENDCLASS.
Nested class statement is not supported.
VISIBILITY OF CLASS COMPONENTS
CLASS <classname> DEFINTION.
//components of class
PUBLIC SECTION.
...
PROTECTED SECTION.
...
PRIVATE SECTION.
...
ENDCLASS.
ABAP class does not define default visibility for its components. Hence it is mandatory to define a visibility section in ABAP class definition and each class component must belong to any one of the visibility sections. A class can have PUBLIC SECTION, PROTECTED SECTION and a PRIVATE SECTION.
PUBLIC SECTION – Components under public section can be accessed from within the class as well as from outside the class. Public section component forms the interface of the class.
PRIVATE SECTION – Components under private section are accessible from within the class only.
PROTECTED SECTION – Components under protected section can be accessed from within the class as well as by child classes of the class. It cannot be accessed from outside the class.
ABAP LOCAL CLASS ATTRIBUTES DECLARATION
Declare attributes to contain data for the class objects.
CLASS <classname> DEFINTION.
//components of class
PUBLIC SECTION.
TYPES: ... .
CONSTANTS: ... .
DATA : <var1> TYPE ...,
<var2> LIKE ...,
<var3> TYPE ... READ-ONLY,
<var4> TYPE REF TO ...
...
...
ENDCLASS.
You declare ABAP class attributes the same way as you declare data objects in ABAP program.
Note that an attribute declared with DATA .. READ-ONLY statement can be read from outside class but cannot be changed from outside class. These attributes can only be changed by methods in the same class and can only be declared under PUBLIC SECTION.
You can also have TYPES and CONSTANTS statement in the class definition.
Encapsulation or information hiding is an important aspect of class. Hence it is recommended that class attributes should be declared as PRIVATE components so that they can only be accessed by class methods and not from outside class.
ABAP LOCAL CLASS METHOD DECLARATION
ABAP Local Class definition may or may not have a method. If method declaration is required, follow the below syntax:
METHODS <method name> [IMPORTING <ip1> TYPE <type> [OPTIONAL|DEFAULT <default value>].....]
[EXPORTING <ep1> TYPE <type> [OPTIONAL|DEFAULT <default value>].....]
[CHANGING <cp1> TYPE <type> [OPTIONAL|DEFAULT <default value>].....]
[RETURNING <rp> TYPE <type> [OPTIONAL|DEFAULT <default value>]]
[{RAISING|EXCEPTIONS} exc1 exc2 ...].
You can declare a method using METHODS statement and can have IMPORTING, EXPORTING, CHANGING, RETURNING parameters.
Remember that methods declared in class definition has to be implemented in class implementation.
Functional methods are methods that have a RETURNING parameter. A method that has RETURNING parameter cannot have EXPORTING and CHANGING parameters. A method can have only one RETURNING parameter.
Later in the course we will learn about event handler methods that can have only IMPORTING parameters.
The method parameters are by default pass by reference. To make them pass by value specify them as VALUE(<ep1>).
To mark an input parameter ( IMPORTING and CHANGING) as optional, use the OPTIONAL or DEFAULT addition.
In order to make method support SY-SUBRC return value, you must define EXCEPTIONS in the method signature, used for the error conditions with the MESSAGE statement. If you want to allow class-based exceptions, use the RAISING addition. Remember that you can define either a EXCEPTIONS or a RAISING addition but not both.
SYNTAX TO CREATE OBJECTS
As we know that class definition is a blueprint. And we have to create instances of the class, known as Objects. We can create any number of objects of a class.
Object creation is a two step process. i) Declare reference variable using DATA … TYPE REF TO statement. ii) Instantiate the reference variable using statement CREATE OBJECT.
Instance components of a class can only be accessed by the reference object. Use CREATE OBJECT statement after START-OF-SELECTION event only.
DATA <reference name> TYPE REF TO <class name>.
CREATE OBJECT <reference name>.
Every object instantiated gets its own memory space in program’s main memory. The reference variable holds the reference to the memory. Thus the reference variable is a pointer to the instance of the class (the memory space). Multiple reference variables pointing to the same object is permitted.
Garbage Collector – The job of garbage collector is to free up memory. If no reference is pointing to an object, it will be removed by the garbage collector.
SYNTAX TO CALL INSTANCE ATTRIBUTES AND INSTANCE METHODS
Use statement obref->attribute to call Instance Attributes. Use statement CALL METHOD objref->methodname to call instance methods. Here CALL METHOD is optional, and you may drop it.
OOABAP LOCAL CLASS EXAMPLES
ABAP LOCAL CLASS EXAMPLE 1
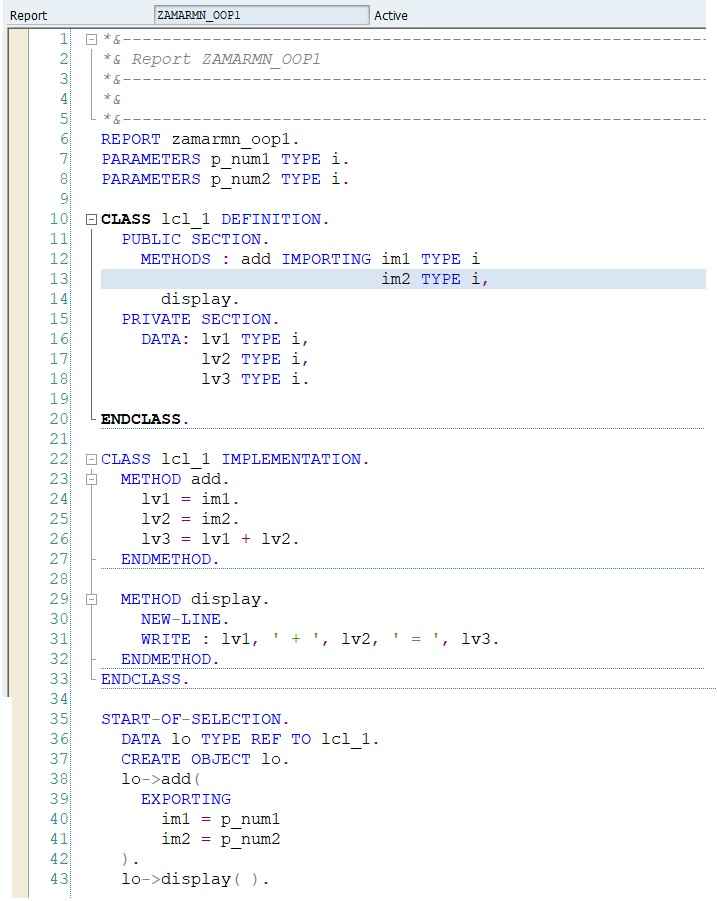
ABAP LOCAL CLASS EXAMPLE 2
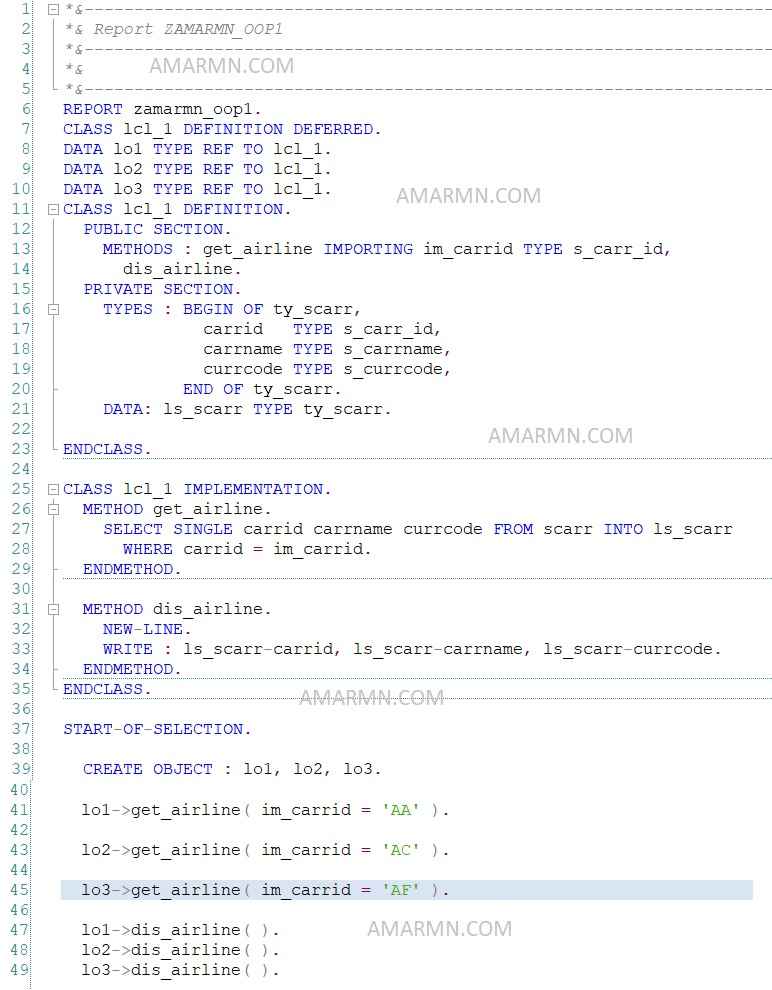