In this lesson we will learn what is function module, how to call function module in ABAP program as well as handle exceptions. In another lesson we will learn how to create a custom function module.
Function modules are procedural blocks stored centrally in the global Function Library of the SAP system and used to encapsulate a functionality. Every function module belongs to a function group. Function Modules are called in an ABAP program using CALL FUNCTION statement.
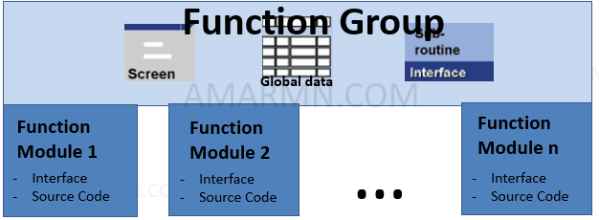
Interface of function module: a function module can have the following types of parameters
- import parameter
- export parameter
- changing parameter
- tables parameter
- exceptions
When a program calls a function module, the entire function group to which the function module belongs is loaded in the working memory of the calling program. A function module can be executed and tested as a standalone object.
SAP system has many standard function modules and we should know how to call a function module in the ABAP program.
Use Pattern button to call function module in an ABAP program
- Place cursor at the line where you want to call the function module
- Choose Pattern button and enter function module name in the new window. Click Continue.
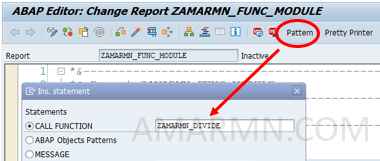
- The function module is called in the ABAP program with complete syntax, i.e interface parameters as well as exceptions.
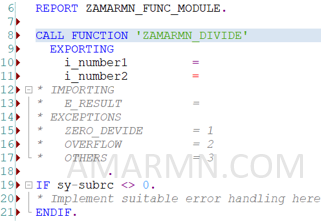
- Let’s complete the ABAP program by passing data object to function module interface parameter as well as handle the exception.
- The function module will throw an exception if the input numbers are out of range or if divide by zero is done
*&---------------------------------------------------------------------*
*& Report ZAMARMN_FUNC_MODULE
*&---------------------------------------------------------------------*
*&
*&---------------------------------------------------------------------*
REPORT zamarmn_func_module.
DATA : lv_num1 TYPE i,
lv_num2 TYPE i,
lv_res TYPE i.
lv_num1 = 100.
lv_num2 = 5.
CALL FUNCTION 'ZAMARMN_DIVIDE'
EXPORTING
i_number1 = lv_num1
i_number2 = lv_num2
IMPORTING
e_result = lv_res
EXCEPTIONS
zero_devide = 1
overflow = 2
OTHERS = 3.
IF sy-subrc <> 0.
* Implement suitable error handling here
CASE sy-subrc.
WHEN 1.
WRITE : 'Division by zero is not allowed'.
WHEN 2.
WRITE : 'Number out of range'.
WHEN OTHERS.
WRITE : 'Division not completed'.
ENDCASE.
ELSE.
NEW-LINE.
WRITE : lv_num1, ' / ', lv_num2, '. Output is:', lv_res.
ENDIF.
Output is :
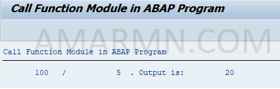