Subroutines are procedural blocks within an ABAP program, used for local modularization. Subroutines are called using statement PERFORM and defined using statement FORM.. ENDFORM.
We can define subroutine in an ABAP program and call the subroutine from the same program (internal subroutine) of some another program ( external subroutine ). It is advised not to use external subroutines, so we will focus on internal subroutines for local program modularization.
Primary function of Subroutines is to encapsulate functions and logic within an ABAP program.
As subroutines are local to program, they can access global data of the main program.
Subroutines should be called first and defined later in the ABAP program. A Subroutine definition is placed at the end of the program as any statement after subroutine definition will not be executed. Subroutines cannot be nested.
A subroutine can be exited using EXIT or CHECK statement. Use EXIT to immediately exit from subroutine. Use CHECK to execute statements following CHECK condition when CHECK statement condition is true.
How to define subroutines
Syntax of subroutine definition
FORM <subroutinename> USING VALUE <ip1> TYPE/LIKE <datatype> "call by value
[VALUE] <ip2> TYPE/LIKE <datatype>
...
CHANGING VALUE <op1> TYPE/LIKE <datatype> "call by value and result
...
<opn> TYPE/LIKE <datatype>. "call by reference
A subroutine can have USING and CHANGING parameters. Parameters of subroutines are by default pass by reference. Use VALUE to make parameters pass by value. Notice that for call by reference, USING and CHANGING are equivalent and server the purpose of documentation. But, for identification purpose, you must define input parameters as USING and output parameters as CHANGING.
The way main program variables are passed to the formal parameters of the subroutine is called the pass type and is specified for each parameter in the subroutine interface. Pass type specification of subroutines is:
- call by value
- call by value and result
- call by reference
You can type subroutine parameter as a generic type using TYPE ANY.
How to call subroutine
PERFORM <subroutinename> USING <actualparameter> ...
CHANGING <actualparameter> ...
.
Subroutines are called using PERFORM statement.
Subroutine example:
*&---------------------------------------------------------------------*
*& Report ZAMIT_SUBROUTINES
*&---------------------------------------------------------------------*
*&
*&---------------------------------------------------------------------*
REPORT zamit_subroutines.
DATA lv_res TYPE i.
DATA: lv_inp1 TYPE i VALUE 10,
lv_inp2 TYPE i VALUE 20.
*calling the subroutine
PERFORM add_by_value USING lv_inp1 lv_inp2 CHANGING lv_res.
PERFORM display.
PERFORM add_by_ref USING lv_inp1 lv_inp2 CHANGING lv_res.
PERFORM display.
*&---------------------------------------------------------------------*
*& Form ADD_BY_VALUE
*&---------------------------------------------------------------------*
* text
*----------------------------------------------------------------------*
* -->P_P_INP1 text
* -->P_P_INP2 text
* <--P_LV_RES text
*----------------------------------------------------------------------*
FORM add_by_value USING VALUE(p_iv1) TYPE i
VALUE(p_iv2) TYPE i
CHANGING VALUE(p_ov1) TYPE i.
p_ov1 = p_iv1 + p_iv2.
p_iv1 = 11.
p_iv2 = 12.
ENDFORM.
*&---------------------------------------------------------------------*
*& Form DISPLAY
*&---------------------------------------------------------------------*
* text
*----------------------------------------------------------------------*
* --> p1 text
* <-- p2 text
*----------------------------------------------------------------------*
FORM display .
NEW-LINE.
WRITE : lv_inp1, ' + ', lv_inp2, ' = ', lv_res.
ENDFORM.
*&---------------------------------------------------------------------*
*& Form ADD_BY_REF
*&---------------------------------------------------------------------*
* text
*----------------------------------------------------------------------*
* -->P_LV_INP1 text
* -->P_LV_INP2 text
* <--P_LV_RES text
*----------------------------------------------------------------------*
FORM add_by_ref USING p_lv_inp1
p_lv_inp2
CHANGING p_lv_res.
p_lv_res = p_lv_inp1 + p_lv_inp2.
p_lv_inp1 = 11.
p_lv_inp2 = 12.
ENDFORM.
The system throws a warning message in syntax check.
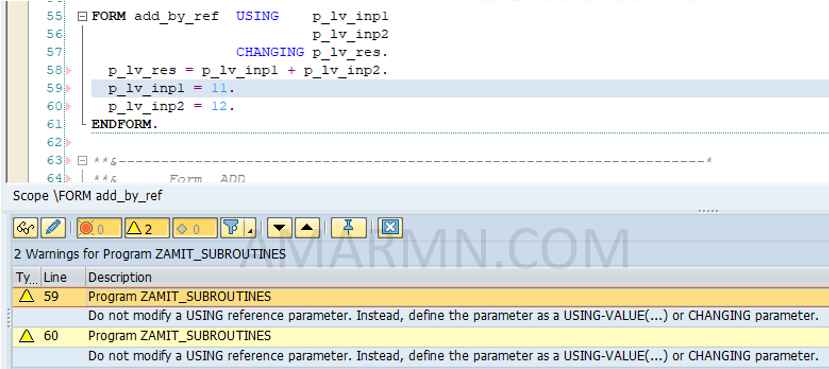
Output:
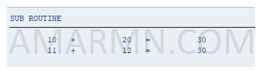