With element, the binding context of an UI element is bound to a specific object in the model data. This creates relative binding within the control and all of its child control are resolved relative to the specific object.
Element binding is specially useful in implementing master/detail scenarios.
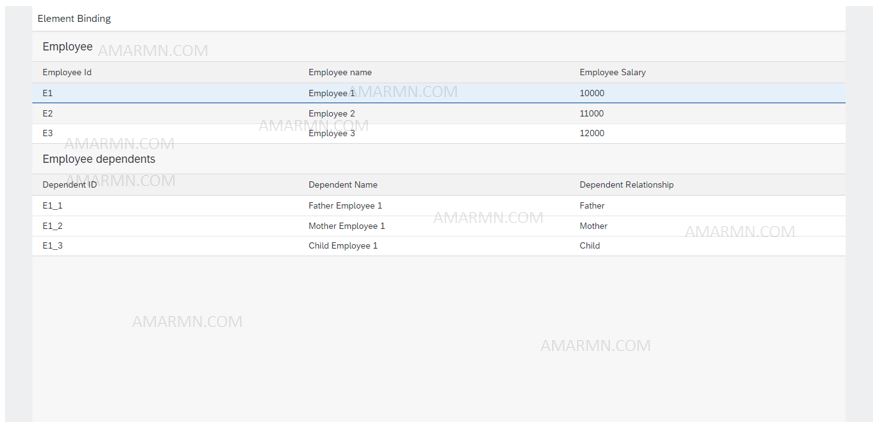
In this SAPUI5 example we use element binding to implement master-detail scenario. The first table displays employees. When an employee row is clicked in the master table, the child table displays its dependents.
Step 1 – Create a data.json file under the model folder.
{
"employeedata": [
{
"employee": {
"empId": "E1",
"empName": "Employee 1",
"empSalary": "10000"
},
"dependents": [
{
"depId": "E1_1",
"depName": "Father Employee 1",
"depRelationship": "Father"
},
{
"depId": "E1_2",
"depName": "Mother Employee 1",
"depRelationship": "Mother"
},
{
"depId": "E1_3",
"depName": "Child Employee 1",
"depRelationship": "Child"
}
]
},
{
"employee": {
"empId": "E2",
"empName": "Employee 2",
"empSalary": "11000"
},
"dependents": [
{
"depId": "E2_1",
"depName": "Father Employee 2",
"depRelationship": "Father"
},
{
"depId": "E2_2",
"depName": "Mother Employee 2",
"depRelationship": "Mother"
}
]
},
{
"employee": {
"empId": "E3",
"empName": "Employee 3",
"empSalary": "12000"
},
"dependents": [
{
"depId": "E3_1",
"depName": "Father Employee 3",
"depRelationship": "Father"
},
{
"depId": "E3_2",
"depName": "Mother Employee 3",
"depRelationship": "Mother"
},
{
"depId": "E3_3",
"depName": "Child 1 Employee 3",
"depRelationship": "Child"
},
{
"depId": "E3_4",
"depName": "Child 2 Employee 3",
"depRelationship": "Child"
},
{
"depId": "E3_5",
"depName": "Child 3 Employee 3",
"depRelationship": "Child"
}
]
}
]
}
Step 2 – Code the view1.view.xml
<mvc:View
controllerName="amarmn.sapui5elementbinding.controller.View1"
xmlns:mvc="sap.ui.core.mvc"
displayBlock="true"
xmlns="sap.m"
>
<Shell id="shell">
<App id="app">
<pages>
<Page id="page" title="{i18n>title}">
<content>
<Table id="_IDGenTable1" items="{/employeedata}" headerText="Employee" mode="SingleSelectMaster"
selectionChange="onEmployeeChange">
<columns>
<Column id="_IDGenColumn1">
<header>
<Text id="_IDGenText1" text="Employee Id"/>
</header>
</Column>
<Column id="_IDGenColumn2">
<header>
<Text id="_IDGenText2" text="Employee name"/>
</header>
</Column>
<Column id="_IDGenColumn3">
<header>
<Text id="_IDGenText3" text="Employee Salary"/>
</header>
</Column>
</columns>
<items>
<ColumnListItem id="_IDGenColumnListItem1">
<cells>
<Text id="_IDGenText5" text="{employee/empId}"/>
<Text id="_IDGenText6" text="{employee/empName}"/>
<Text id="_IDGenText7" text="{employee/empSalary}"/>
</cells>
</ColumnListItem>
</items>
</Table>
<Table id="idDepTable" items="{dependents}" headerText="Employee dependents">
<columns>
<Column id="_IDGenColumn5">
<header>
<Text id="_IDGenText9" text="Dependent ID"/>
</header>
</Column>
<Column id="_IDGenColumn6">
<header>
<Text id="_IDGenText10" text="Dependent Name"/>
</header>
</Column>
<Column id="_IDGenColumn7">
<header>
<Text id="_IDGenText11" text="Dependent Relationship"/>
</header>
</Column>
</columns>
<items>
<ColumnListItem id="_IDGenColumnListItem2">
<cells>
<Text id="_IDGenText12" text="{depId}"/>
<Text id="_IDGenText13" text="{depName}"/>
<Text id="_IDGenText14" text="{depRelationship}"/>
</cells>
</ColumnListItem>
</items>
</Table>
</content>
</Page>
</pages>
</App>
</Shell>
</mvc:View>
Step 3 – view1.controller.js
sap.ui.define([
"sap/ui/core/mvc/Controller"
],
/**
* @param {typeof sap.ui.core.mvc.Controller} Controller
*/
function (Controller) {
"use strict";
return Controller.extend("amarmn.sapui5elementbinding.controller.View1", {
onInit: function () {
var oModel = new sap.ui.model.json.JSONModel;
oModel.loadData("model/data.json");
this.getView().setModel(oModel);
},
onEmployeeChange: function (oEvent) {
var oListItem = oEvent.getParameter("listItem");
var sPath = oListItem.getBindingContext().getPath();
var oTable = this.byId("idDepTable");
oTable.bindElement(sPath);
}
});
});