Use the sap.ui.define() to define SAPUI5 modules. Modules helps in modularizing applications JavaScript code into smaller reusable code blocks.
In this SAPUI5 example we see how to create SAPUI5 modules.
Step 1 – In the SAPUI5 project, create a folder called utility, under the webapp folder. Under utility folder, create file Formatter.js and MessageManager.js
Step 2 – Code the Formatter.js file
sap.ui.define([],
function () {
"use strict";
return {
capitalizeFirstLetter: function (sValue) {
return sValue.charAt(0).toUpperCase() + sValue.slice(1);
}
}
});
Step 3 – Code the MessageManager.js file
Here we are defining a function displayMessage. Inside the displayMessage we are calling the MessageBox as well as function of Formatter.js file.
sap.ui.define(["sap/m/MessageBox",
"kumar/zamitsapui51/utility/Formatter"
],
function (MessageBox, Formatter) {
"use strict";
return {
openMessageBox: function (sMsg, sTitle) {
MessageBox.show(
Formatter.capitalizeFirstLetter(sMsg), {
title: Formatter.capitalizeFirstLetter(sTitle)
});
}
}
});
Step 4 – Create a button in view.xml file
<Page id="page" title="{i18n>title}">
<content>
<Button text="Press the Button" press="onButtonPress"/>
</content>
</Page>
Step 5 – Code onButtonPress function in Controller.js file
sap.ui.define([
"sap/ui/core/mvc/Controller",
"kumar/zamitsapui51/utility/MessageManager"
],
function (Controller,MessageManager) {
"use strict";
return Controller.extend("kumar.zamitsapui51.controller.View1", {
onInit: function () {
},
onButtonPress : function() {
MessageManager.openMessageBox("the button was pressed!!!!!!!","message box");
}
});
});
Test the Application.
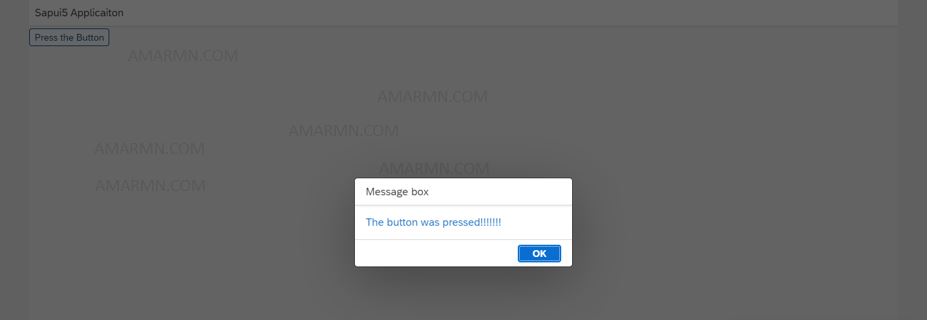